Objects in javascript are two-faced. They combine two important functional.
The first is an associative array: a structure suitable for storing any data. In this chapter, we will look at using objects as arrays.
The second is language capabilities for object-oriented programming. We will study these possibilities in the subsequent sections of the textbook.
Associative arrays
An associative array is a data structure in which you can store any data in key-value format.
It can be easily represented as a cabinet with signed drawers. All data is stored in boxes. By name, you can easily find the box and take the value that lies in it.
Unlike real cabinets, you can add new named “boxes” to an associative array at any time or delete existing ones. Next we will see examples of how this is done.
By the way, in other programming languages such data structure is also called “dictionary” and “hash” .
Creating objects
An empty object ( “empty cabinet” ) can be created with one of two syntaxes:
Usually everyone uses the syntax (2)
, because he is shorter.
Object operations
An object can hold any values that are called object properties . Properties are accessed by property name (sometimes referred to as “by key” ).
For example, create a person
object to store information about a person:
Basic operations with objects are:
- Assigning properties by key.
- Reading properties by key.
- Deleting property by key.
To access the properties, use the “through point” entry, of the объект.свойство
type:
Next operation:
- Check for the existence of a property with a specific key.
For example, there is a person
object, and you need to check if the age
property exists in it.
To check for existence, there is an in
operator. Its syntax is "prop" in obj
, and the name of the property is in the form of a string, for example:
3 | if ( "age" in person ) { |
4 | alert( "Свойство age существует!" ); |
However, another way is often used - comparing the value with undefined
.
The fact is that in JavaScript you can refer to any property of the object, even if it does not exist . There will be no error.
But if the property does not exist, then the special value undefined
will be returned:
Thus, we can easily check the existence of a property — by getting it and comparing it with undefined
:
There are two means for checking the presence of a property in an object: the first is the in
operator, the second is to get it and compare it with undefined
.
They are almost identical, but there is one small difference.
The fact is that it is technically possible that the property is and equals undefined
:
... At the same time, as can be seen from the code, with a simple comparison, the presence of such a property will be indistinguishable from its absence.
But the in
operator guarantees the correct result:
As a rule, in the code we will not assign undefined
, so that both checks work correctly. And as a value denoting uncertainty and uncertainty, we will use null
.
Access through square brackets
There is an alternative syntax for working with properties, using the brackets объект['свойство']
:
In square brackets you can pass a variable:
obj[key]
uses the value of the key
variable as the property name:
The person['age']
and person.age
identical. On the other hand, if the name of a property is stored in a variable ( var key = "age"
), then the only way to access it is with square brackets person[key]
.
Appeal through a dot is used if we already know the name of the property at the stage of writing the program. And if it is determined in the course of execution, for example, entered by a visitor and written into a variable, then the only choice is square brackets.
- The name of the property when accessing "through the point" imposed syntactical restrictions - about the same as the variable name. It cannot begin with a number, contain spaces, etc.
- When accessed through square brackets, you can use any string.
For example:
person[ 'день рождения!' ] = '18.04.1982' ; |
In both cases, the property name must be a string . If a value of another type is used, JavaScript will automatically string it.
Properties ad
An object can be filled with values when created by specifying them in curly braces: { ключ1: значение1, ключ2: значение2, ... }
.
This syntax is called literal (the original is literal ), for example:
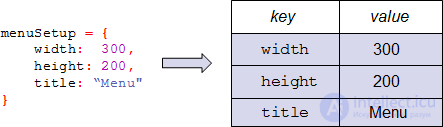
The following two code fragments create the same object:
10 | menuSetup.width = 300; |
11 | menuSetup.height = 200; |
12 | menuSetup.title = 'Menu' ; |
Property names can be listed in quotes or without quotes if they satisfy the constraints for variable names.
For example:
4 | "мама мыла раму" : true |
Importance: 3
3 | user.surname = "Петров" ; |
[Open task in new window]
Nested objects in the ad
The property value can be an object:
13 | alert( user.size.top ) |
Here, the value of the size
property is the {top: 90, middle: 60, bottom: 90 }
object.
For debugging purposes, sometimes you want to display the entire object. Firefox has a non-standard toSource method for this.
In other browsers it is not, but the object can be viewed through the developer tools: debugger or console.log
.
Enumerate properties and values
To iterate over all properties from an object, the loop is used for the properties for..in
.. This is a special syntactic construct that does not work like a regular for (i=0;i<n;i++)
loop for (i=0;i<n;i++)
.
Syntax:
In this case, the property names will be sequentially written in the key
.
Variables can be declared directly in the loop:
So sometimes they write for short code.
For example:
Of course, instead of key
can be any other variable name.
The number of properties in the object
There is no one method that would return the number of properties. Cross-browser method is to loop through the properties and count:
1 | function getKeysCount(obj) { |
3 | for ( var key in obj) { |
And this is how the function of checking for “emptiness” looks like:
1 | function isEmpty(obj) { |
2 | for ( var key in obj) { |
The order of the properties
Are the properties ordered in objects? In theory, i.e. according to the specification - no. In practice, everything is more complicated.
Browsers adhere to an important agreement on how to iterate through properties. It, though not spelled out in the standard, but rather reliably, because a lot of existing code depends on it.
- IE <9 browsers, Firefox, Safari scroll through the keys in the same order in which the properties were assigned.
- Opera, modern IE, Chrome guarantee order preservation only for string keys. Numeric keys are sorted and go to string.
That is, if the properties in the object are string, then such an object is ordered. Properties will be sorted in the order of their assignment:
Consider now the case when the keys are “numerical”. As we know, objects in JavaScript can have keys only as strings, but if the string is in integer form, some interpreters recognize this and process such keys differently.
For example, consider an object that specifies a list of options for selecting a country:
We want to bring these options to the visitor in the same order, that is, "Tanzania" at the beginning, and "USA" - at the end (this is if our office is located in Zanzibar, then this order will be most relevant).
However, when browsing them through for..in
some browsers (IE9 +, Chrome, Opera) will display the keys not in the order in which they are specified, but in a sorted order :
Breaking the order arose because the keys are numerical. To bypass it, you can use a small hack, which is that all keys are artificially made lowercase. For example, add them the first additional character '+'
.
In this case, the browser will consider the keys as string and preserve the brute force order:
Transfer by reference
Common values: strings, numbers, booleans, null/undefined
copied "by value" .
This means that if the message
variable stores the value "Привет"
and it is copied with the phrase = message
, then the value is copied, and we will have two variables, each of which stores the value: "Привет"
. "Привет"
Objects are assigned and transmitted "by reference . "
The variable does not store the object itself, but a link to its place in memory. To better understand this - comparable to ordinary variables.
For example:

And this is what the user = { name: "Вася" }
variable looks like.
Attention: the object is outside the variable . In a variable, there is only a reference (“the address of the place in memory where the object lies”).
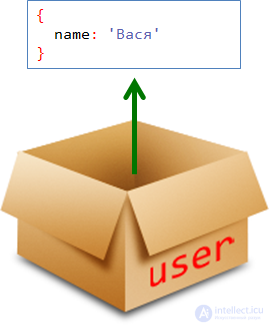
When copying a variable with an object, this link is copied, and the object still remains in a single copy.
It turns out that several variables refer to the same object:
var user = { name: "Вася" }; |
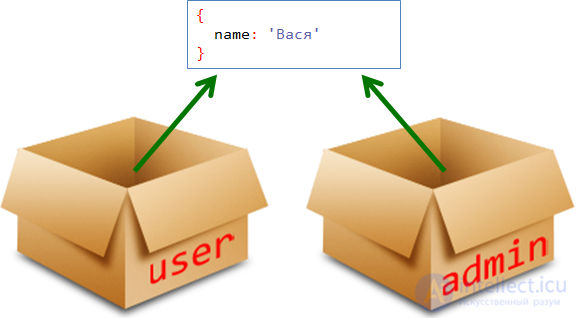
Since the object is only one, in whatever variable it is not changed - this is reflected in the others:
Another analogy: the variable to which the object is assigned, in fact, does not store the data itself, but the code to the safe where it is stored.
When passing it to a function, this code is copied to local variables, so that the variable can make changes to these data.
So, when transferring an object somewhere, only the link to it is copied.
To copy the data itself, you need to get it from the object and copy it at the primitive level.
Like that:
Importance: 5
[Open task in new window]
Compact representation of objects
This section refers to the internal structure of the data structure and requires special knowledge. It is not required to read.
An object, as described, takes a lot of memory space, for example:
It contains information about the
name
property and its string value, as well as the
age
property and its numerical value. Imagine that there are many such objects.
It turns out that the information on the names of the properties name
and age
duplicated in each object.
To avoid this, browsers use a special "compact representation of objects."
When creating a set of objects of the same type (with the same fields), the interpreter remembers how many fields it has and what they are, and stores this data in a separate structure. And the object itself is in the form of a continuous data array.
For example, for objects of the form {name: "Вася", age: 25}
a structure will be created that describes this kind of objects: "the name
string, then the age
", and the objects themselves will be represented in the data: Вася25
. And instead of a string, there will usually be a pointer to it.
The browser for accessing the property will know from the structure where it lies and will go to the desired position in the data. It is very fast, and saves memory, when there are many similar objects in a single structure description.
What happens if a new field is added to the object? For example:
In this case, the browser looks to see if there is already a structure under which such an object fits (“string name
, integer age
, logical admin
”). If not, it is created. The changed object data is bound to it.
Details of the application and implementation of this storage method vary from browser to browser. Apply additional optimizations.
You can learn more about the internal structure of types, for example, from the presentation Know Your Engines (Velocity 2011).
Total
Objects are associative arrays with additional features:
- Access to the elements is provided:
- Directly by key
obj.prop = 5
- Through the variable in which the key is stored:
- Deleting keys:
delete obj.name
. - Loop by keys:
for (key in obj)
, the search order corresponds to the order of declarations for string keys, and for numeric keys it depends on the browser. - The existence of a property can be checked by the
in
: if ("prop" in obj)
operator, as a rule, it works and a simple if (obj.prop !== undefined)
comparison if (obj.prop !== undefined)
. - The variable does not store the object itself, but a link to it. Copying such a variable and passing it to a function duplicate the link, but the object remains alone.
Comments
To leave a comment
Scripting client side JavaScript, jqvery, BackBone
Terms: Scripting client side JavaScript, jqvery, BackBone