Often we need to repeat the same action in many parts of the program.
For example, it is necessary to display a message when greeting a visitor, when a visitor leaves the site, somewhere else.
In order not to repeat the same code in many places, functions are invented. Functions are the basic building blocks of the program.
Examples of built-in functions you have already seen are alert(message)
, prompt(message, default)
and confirm(question)
. But you can create your own.
Announcement
Example function declaration:
alert( 'Привет всем присутствующим!' ); |
First comes the function
keyword, after it is the name of the function , then the list of parameters in brackets (in the example above it is empty) and the function body — the code that is executed when it is called.
The declared function is available by name, for example:
This code will display the message twice. The main purpose of creating functions is already visible here : getting rid of code duplication .
If you need to change the message or the method of its output, it is enough to change it in one place: in the function that outputs it.
Local variables
The function may contain local variables declared via var
. Such variables are visible only inside the function:
if/else
, switch
, for
, while
, do..while
blocks do not affect the scope of variables.
When a variable is declared in such blocks, it will still be visible in the entire function.
For example:
2 | for ( var i=0; i<3; i++) { |
It doesn't matter where exactly in the function and how many times the variable is declared. Any announcement is triggered once and applies to the entire function.
Variable declarations in the example above can be moved up, this will not affect anything:
External variables
The function may refer to an external variable, for example:
Access is possible not only for reading, but also for writing. In this case, since the variable is external, the changes will be visible outside the function:
Of course, if inside a function, in line (1)
, its own local variable var userName
were declared, then all calls would use it, and the external variable would remain unchanged.
Variables declared at the level of the entire script are called “global variables” .
Make global only those variables that really have a common meaning for your project.
Let each function work “in its own sandbox”.
In the old JavaScript standard, there was the possibility of implicitly declaring variables by assigning a value.
For example:
In the code above, the message
variable is not declared anywhere, but is immediately assigned. Most likely, the programmer simply forgot to install var
.
In the modern JavaScript standard, such an assignment is prohibited, and in the old one, which works in browsers by default, the variable will be created automatically, and in the example above it is created not in the function, but at the level of the entire script.
Avoid this.
Here, the danger is not even in the automatic creation of a variable, but in the fact that global variables should be used when “common script” parameters are really needed.
Lost var
in one place, then another - as a result, two functions unexpectedly changed the same global variable for each other.
In the future, when we get better acquainted with the basics of JavaScript, in the chapter Closures, functions from the inside, we will take a closer look at the internal mechanisms of variables and functions.
Options
When you call a function, you can transfer data to it that it uses at its discretion.
For example, this code displays two messages:
Parameters are copied to local function variables .
In the example below, the change from
in the string (1)
will not affect the value of the external variable from
(2)
, since The copy of the value was changed:
Default Arguments
The function can be called with any number of arguments.
For example, the showMessage(from, text)
message display function showMessage(from, text)
can be called with one argument:
If the parameter is not passed during the call, it is considered to be equal to undefined
.
Such a situation can be caught and assigned the value “by default”:
When declaring a function, optional arguments are usually placed at the end of the list.
To specify the value of "default", that is, one that is used if the argument is not specified, two methods are used:
- You can check whether the argument is
undefined
, and if so, write the default value in it. This method is demonstrated in the example above. - Use operator
||
: The second method assumes that the argument is absent if an empty string is passed, 0
, or in general any value that is false
in the boolean form.
If there are more arguments than you need, for example showMessage("Маша", "привет", 1, 2, 3)
, then there will be no error. But since no parameters are provided for “extra” arguments, they can only be accessed through the special arguments
object, which we will look at in the Pseudo-array of arguments chapter.
Feature Declaration Style
In the function declaration there are rules for the arrangement of spaces. They are marked with arrows:
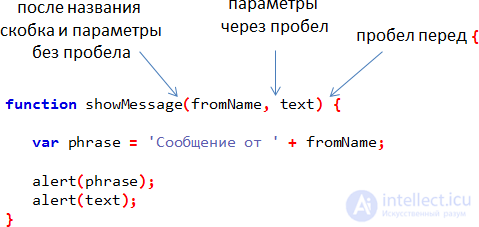
Of course, you can put spaces in a different way, but these rules are used in most JavaScript frameworks.
Return value
A function can return a result that will be passed to the code that called it.
For example, create the calcD
function, which will return the discriminant of a quadratic equation using the formula b 2 - 4ac
:
To return a value, use the return
directive.
It can be anywhere in the function. As soon as control reaches it, the function ends and the value is transferred back.
There can be several return
calls, for example:
Importance: 4
Both options function the same, there is no difference.
[Open task in new window]
Importance: 4
Using the operator '?'
:
return (age > 18) ? true : confirm( 'Родители разрешили?' ); |
Using the operator ||
(shortest option):
return (age > 18) || confirm( (age > 18) || confirm( 'Родители разрешили?' ); |
[Open task in new window]
The return
directive can also be used without a value to terminate and exit a function.
For example:
1 | function showMovie(age) { |
6 | alert( "Фильм не для всех" ); |
In the code above, if the if worked, then the string (*)
and all the code under it will never be executed, since return
completes the execution of the function.
In the case when the function did not return a value or the return
was without arguments, it is considered that it returned undefined
:
Please note there is no error. Just returning undefined
.
Another example, this time with return
with no argument:
Name selection
The function name follows the same rules as the variable name. The main difference is that it should be a verb, since function is action.
As a rule, verb prefixes are used to indicate the general nature of the action, followed by clarification.
Functions that start with "show"
- show something:
Functions beginning with "get"
- receive, etc.:
This is very convenient, because looking at a function, we’re about what it does, even if the function was written by a completely different person, and in some cases, and what kind of value it returns.
A function must do only what is explicitly implied by its name. And it should be one action.
If it is complex and implies sub-actions - maybe it makes sense to isolate them into separate functions? Often it makes sense to better structure the code.
... But the most important thing is that there should be nothing in the function except the action itself and the sub-actions that are inseparably connected with it.
For example, the data validation function (say, "validate"
) should not show an error message. Her action is to check.
Names of functions that are used very often sometimes make it ultrashort.
For example, in the jQuery framework there is a $
function, in the Prototype framework the $$
function, and in the Underscore library the function with the name of a single underscore symbol _
very actively used.
Total
Function declaration:
function имя(параметры, через, запятую) { |
- The values passed are copied to the function parameters and become local variables.
- Function parameters are its local variables.
- You can declare new local variables with
var
. - The value is returned by the operator
return ...
- Calling
return
immediately terminates the function. - If
return;
called without a value, or the function terminated without return
, then its result is equal to undefined
.
When accessing an undeclared variable, the function will search for an external variable with this name.
If possible, it is recommended to use local variables and parameters:
- This makes the overall execution flow obvious - what is passed to the function and what is the result.
- This prevents possible access conflicts when two functions, possibly written at different times or by different people, unexpectedly use the same external variable.
Function Naming:
- The name of the function should be clear and clearly reflect what it does. After seeing her call in the code, you should immediately understand what she is doing.
- A function is an action, so verbs are usually used for function names.
Functions are the basic building blocks of scripts. We will repeatedly return to them and study more and more deeply.
Importance: 1
Decision
[Open task in new window]
Importance: 4
Solution: tutorial / intro / pow.html
Comments
To leave a comment
Scripting client side JavaScript, jqvery, BackBone
Terms: Scripting client side JavaScript, jqvery, BackBone