Depending on what you are doing the script, you will need to work with the information.
If this is an electronic store, then this is a goods basket. If the chat - visitors, messages and so on.
Variables are used to store information.
Variable
A variable consists of a name and a dedicated area of memory that corresponds to it .
To declare or, in other words, create a variable, use the var
keyword:
After the declaration, you can write to the variable data:
This data will be stored in the corresponding memory area and will be available later on by name:
For brevity, you can combine a variable declaration and a data record:
Analogy from life
The easiest way to understand a variable is to present it as a “box” for data, with a unique name.
For example, the message
variable is a box that stores the value of "Привет"
:
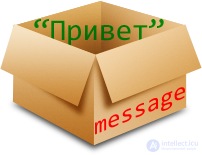
You can put any value in the box, and later change it. The value in a variable can be changed as many times as necessary:
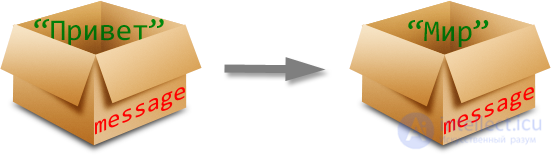
When the value changes, the old contents of the variable are deleted.
There are functional programming languages in which the value of a variable cannot be changed.
In such languages, once put the value in the box - and it is stored there forever, neither deleted nor changed. And you need to save something else - if you please, create a new box (declare a new variable), reuse is impossible.
In appearance, it is not very convenient, but, oddly enough, it is quite possible to successfully program in such languages. The study of some functional language is recommended to expand the horizons. An excellent candidate for this is the Erlang language.
Copying values
Variables in JavaScript can store not only strings, but also other data, such as numbers.
Let's declare two variables, put a string in one, and a number in the other.
As you can see, the variable does not matter what to store:
The value can be copied from one variable to another.
2 | var message = 'Привет' ; |
The value from
num
overwrites the current in
message
.
In the "box" message
value changes :
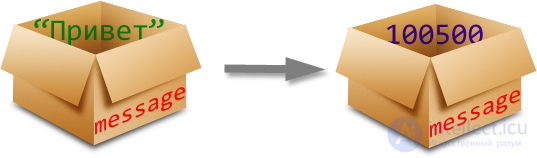
After this assignment, in both num
and message
boxes is the same value 100500
.
Importance of the var
directive
In JavaScript, you can create a variable without var
, just assign a value to it:
Technically, this will not cause an error, but you shouldn’t do it anyway.
Always define variables through var
. This is a good programming tone and helps to avoid mistakes.
Open an example in IE in a new window :
03 | < meta http-equiv = "X-UA-Compatible" content = "IE=8" > |
06 | < div id = "test" ></ div > |
Open code in new window
The value does not appear, there will be an error. If debugging is enabled in IE or the development panel is open, you will see it.
The fact is that almost all modern browsers create variables for elements that have an id
.
Variable test
in them in any case exists, run, for example:
.. But in IE <9 such a variable cannot be changed.
All will be well if to declare test
, using var
:
The correct code is:
The most "funny" is that this error will only be in IE <9, and only if there is an element with a matching id
on the page.
Such errors are especially fun to fix and debug.
There are also situations where the absence of a var
can lead to errors. I hope you are convinced of the need to always put var
.
Importance: 2
Each line of the solution corresponds to one step of the task:
[Open task in new window]
Constants
A constant is a variable that never changes. As a rule, they are called in capital letters, underlined. For example:
1 | var COLOR_RED = "#F00" ; |
2 | var COLOR_GREEN = "#0F0" ; |
3 | var COLOR_BLUE = "#00F" ; |
4 | var COLOR_ORANGE = "#FF7F00" ; |
Technically, a constant is a regular variable, that is, it can be changed. But we agree not to do this.
Why do we need constants? Why not just use "#F00"
or "#0F0"
?
- First, the constant is a friendly name, unlike the string
"#FF7F00"
. - Secondly, a typo in the string may not be seen, and in the name of the constant it is impossible to miss it - there will be an error during execution.
Constants are used instead of lines and numbers to make the program clearer and to avoid errors.
Comments
To leave a comment
Scripting client side JavaScript, jqvery, BackBone
Terms: Scripting client side JavaScript, jqvery, BackBone